What is MATLAB?
MATLAB is a powerful software application developed and supported by Mathworks
MATLAB is a high level programming language used by engineers, scientists and economists to help solve complex mathematical problems. A MATLAB program is written to “model” a system, for instance a yaw damper on a commercial jet. The characteristics of the system can be investigated by changing the inputs or other parameters (bank angle, sideslip angle) and noting the effects on the outputs (yaw rate, roll rate). Without software this would normally require very tedious repetition of calculations.
MATLAB includes many advanced computational and graphical routines and a user can create his/her own functions, allowing programs to be written for any application of mathematics.
Increasingly, software is replacing hardware in control systems. For instance, MATLAB programs can be written to perform the function of filters for digital signal processing.
MATLAB stands for “matrix laboratory” because it is based on rectangular arrays of numbers called matrices like the one shown below:
The algebra of matrices is similar in some ways to that of single numbers, but there are also many important differences. Bear this in mind if you can't get your program to work! Also, MATLAB programs can only be written successfully if the writer understands the maths behind the program.
MATLAB is “command driven”. This means that the user creates a program by writing a sequence of commands. Each command must conform to strict rules (“syntax”) as must the way the commands link together. MATLAB has extensive help facilities to aid the user and you will get more information about this further on in this learning resource.
Accessing MATLAB
As a UHI student, you have FREE access to MATLAB software download via an institutional license. Click on the link below to start the process of installing a MATLAB license from LIS (Learning and Information Services). This involves contacting help desk in the first instance to request an activation code.
Access MATLAB
https://www.uhi.ac.uk/en/lis/software-downloads/matlab-home-use/
Follow the guidance on the UHI website above, or read it here: Installing MATLAB Student
You may also find this video guide on installing MATLAB useful
Learning Resources
There is a multitude of free resources available to you to help you learn how to use MATLAB basics. In this resource we will show you an introductory video, and offer some practical exercises to get you started on uses of MATLAB for engineering.
Mathworks website also has a number of online courses which do cost and are appropriate for more in depth specialised learning.
However, the resources highlighted below are excellent ways to find out how MATLAB works, using videos and in - software instruction to step you through introductory exercises and we would recommend you undertake one of these before using MATLAB to undertake assessment activities with it on your module.
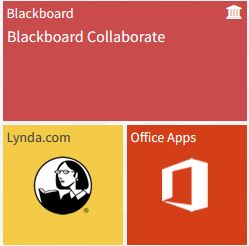
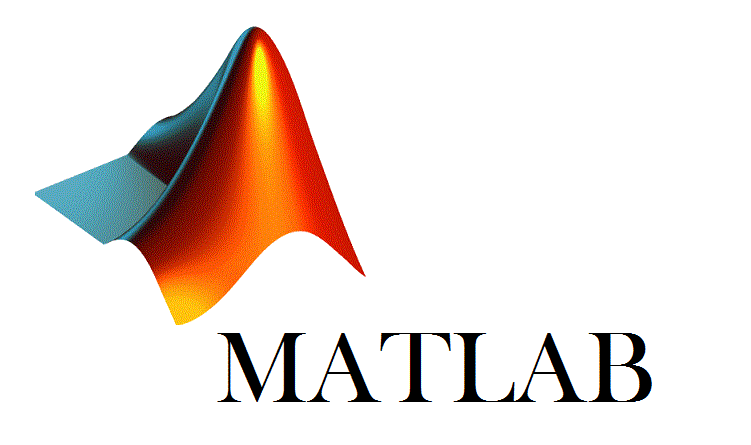
First Look at MATLAB
The videos below are from one of the previous YouTube video channels mentioned, The video on the right is from the MATLAB for Engineers channel and The Complete MATLAB course by Joseph Delgadillo. These give you an idea of how MATLAB looks in operation.
The video on the right in a complete package of over 4 hours which should skip to 7.55s so you can view an introductory video on the MATLAB interface and basic functions. Bear in mind though it was produced in 2016 and working with an older version of MATLAB, whereas the video on the right is from 2018.
MATLAB Onramp
MATLAB Onramp is an free, introductory, step by step course from Mathworks which allows you to work through a series of instructions in MATLAB in your browser to practice using it. You need to have a Mathworks account (and MATLAB license?) to access the course. The tasks you are set are assessed by the program, errors flagged up and hints given if required on the basis of the errors you make. The topics covered include commands, vectors and matrices, importing data, using help, arrays, plotting data, programming and more.
If you learn by doing, you will find this course invaluable!
MATLAB Onramp
You can access MATLAB Onramp training on the Mathworks website: Onramp training
Step Through MATLAB
The following pages will take you through some starter exercises to introduce you to MATLAB use.
Step 1: MATLAB user interface
When you open MATLAB the command window appears:
Commands are entered at the command prompt. Try these:
>> A = [1 2 3; 4 5 6]
>> B = [2 4 6; 4 6 8]
>> A+B
The elements in a matrix are contained within square brackets. Spaces or commas are used to separate elements in a row. Semicolons are used to separate rows. A semicolon (;) can also be used to stop the output of a command appearing on the command window:
>> P = [-0.5 7 2.9 -3.8];
>> q = [1, 0.9, 0];
>> s = [3; -2; 6; -0.5]
>> r = p*s
This program creates a new variable called r which could be used later in the program. Also visible are the current directory and the command history.
M-Files
Step 2: M - Files
When working in the MATLAB command window commands are executed one by one as the Enter key is pressed. However, it is usually better to create a file of all the commands you want in your program. The file can then be saved and opened as required and the program executed whenever you like. This is called an M-file because it is saved with the extension .m, for example “filename.m”. To create or open an M-file use “New” or “Open” on the main menu. There are two types of M-files: script files and functions.
Here is an example of an M-file that can be used to solve the following set of simultaneous equations:
%Solving simultaneous equations
%Write coefficients of variables x, y and z as a matrix
A = [3 1 4;-1 -2 6;2 3 -8];
%Write RHS as column matrix
B = [4; 5.5; -7];
%Find inverse of matrix A
C = inv(A);
%To find solution, multiply inverse of matrix A by B
P = C*B
You can execute the program by clicking on the Run button on the main menu. The output of this program can be seen in the command window:
P =
2.0000
-3.0000
0.2500
MATLAB has worked out that x = 2, y = -3 and z = 0.25.
Notice that the M-file includes remark (comment) statements to help the reader understand the program and follow what it is doing.
In MATLAB remark statements start with a % character. They appear in green and do not affect the execution of a program.
Entering Data
Step 3: Entering data
You may want to use MATLAB to help analyse experimental data. It is tedious and error prone to type the data directly into your program. Instead, MATLAB will read files from other programs such as Notepad. The data is entered into columns in the Notepad file, with columns separated by a tab. It is saved as a text file (.txt). (In Windows, Notepad is found from Start – My programs – Accessories.)
%Load data file
load data1.txt
%Enter input data
input = data1(:,1);
%Enter output data
output = data1(:,2);
This program reads in data contained in the file data1.txt. (For your MATLAB program to find this file it has to be in your current directory. You may have to copy it there.) This chunk of program also creates a variable called “input” from the first column of the data and another variable called “output” from the second column.
Graphs
Step 4: Graphs
MATLAB is very good for plotting graphs. All sorts of sophisticated graphs can be created, including logarithmic, contour and 3-D plots. The following commands create a graph for the function y = cos2x:
%This program plots a graph of a trig function
clear all
%create the independent variable x from 0 to 2pi in steps of 0.1
x = [0 : 0.1 : 2*pi];
%create the depndent variable y
y = cos(2*x);
%plot the graph
plot(x, y)
title('The function y = cos2x')
xlabel('x - angle in radians')
ylabel('y')
The following graph is produced:
Bar charts are also possible:
%This program plots a bar chart for rainfall in Scone
%create the independent variable x for the months
x = [1:12];
%create the dependent variable y for the monthly rainfalls in mm
y = [98 87 102 68 49 40 55 61 75 81 88 93];
%plot the graph
bar(x, y)
title('The rainfall at Scone in 2008')
xlabel('month')
ylabel('rainfall /mm')
Summary
We hope you have found this a useful introduction to MATLAB. To summarise what you should do to start using MATLAB, follow the steps below:
- Get in touch with LIS to install a free UHI license on your own computer – there instruction in this learning resource, or access them at https://www.uhi.ac.uk/en/lis/software-downloads/matlab-home-use/
- There are a few different series of introductory videos that will help you get to grips with using MATLAB. You can find these on Lynda.com, YouTube and the Mathworks website.
- If you want work through a step by step practice of using MATLAB’s basic functions, then undertake the MATLAB Onramp course on the Mathworks website.